parseInt() - Explained with Examples
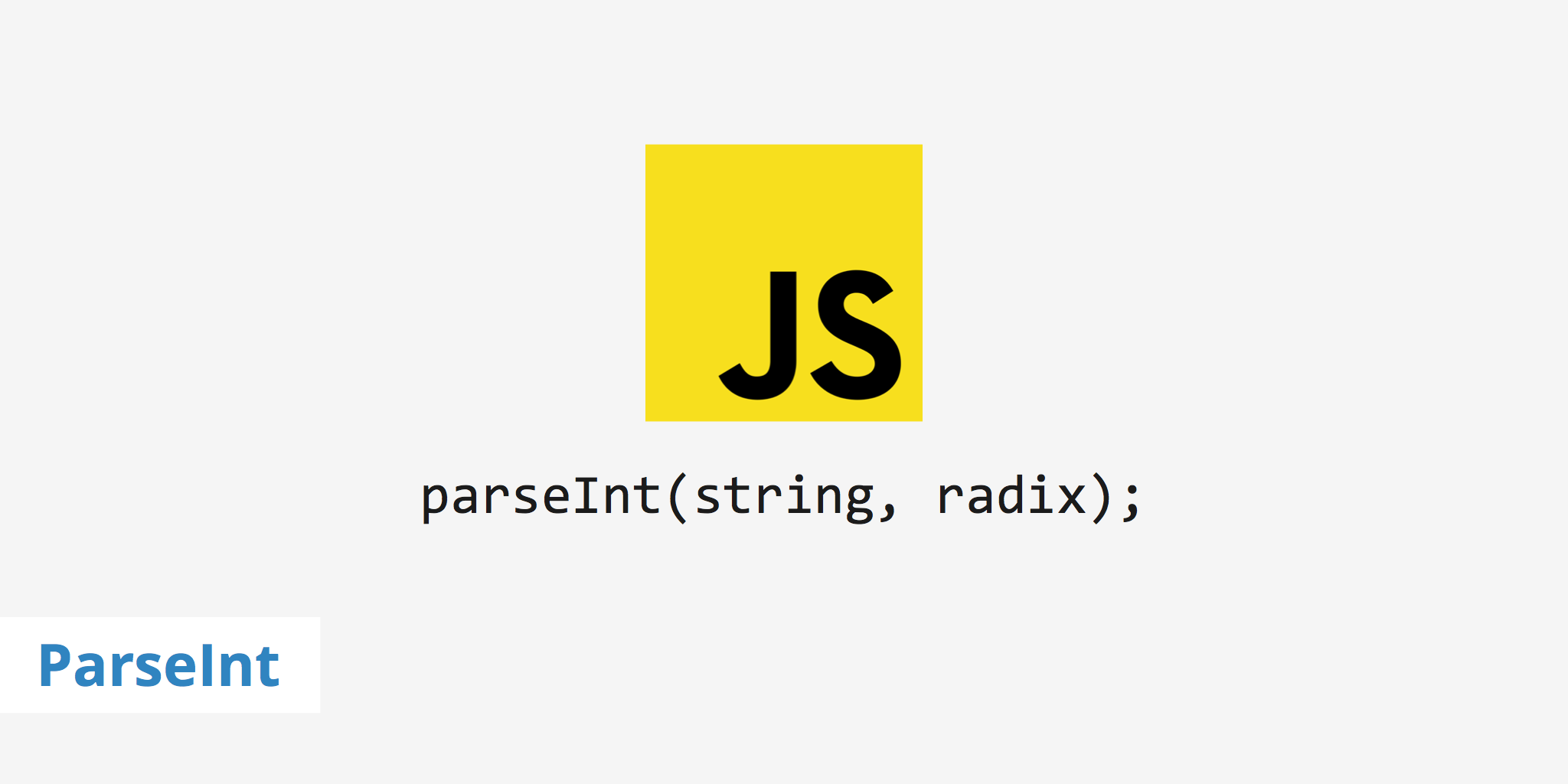
There are many functions one can use in JavaScript. In this post, we'll be covering the parseInt()
function. The goal of this post is to explain what parseInt()
does, how to use it, and provide a few examples of how it works.
What is parseInt()
?
parseInt()
is a JavaScript function that parses a string and returns an integer. It allows you to define two separate parameters and uses the following syntax:
parseInt(string, radix);
string
- The value that is to be parsed. If the argument value is not a string, it will be converted to one using the ToString abstraction method.radix
- The radix represents the numeral system to be used. This can be a value between2
and36
. Although optional, it's recommended to define a radix value to avoid any warnings or confusion. A radix value of10
is commonly used.
How to use parseInt()
You can use parseInt()
by inputting a decimal or string in the first argument and then defining a radix. If the first argument cannot be converted into a number, parseInt()
will return NaN
. If the radix is not defined in a parseInt()
function it will assume the following:
- If the string begins with
0x
or0X
, the radix will be16
(hexadecimal). - If the string begins with
0
, the radix will be8
(octal). This has since been deprecated with ECMAScript 5. - If the string begins with any other value, the radix will be
10
(decimal).
Using parseInt()
is useful in situations where you have a string number like 0500
but want the output integer to be 500
. parseInt()
easily converts your string to a rounded integer. In these cases, simply define your string argument and set the radix to 10
(or if you have a special use-case, another numerical value).
parseInt()
examples
To help provide a clearer representation of how parseInt()
works, check out the examples and results below. For this, we've outlined three octal examples, three decimal examples, and three hexadecimal examples.
Octal
parseInt('10', 8); //returns 8
parseInt('010') //returns 10
parseInt('17', 8); //returns 15
Decimal
parseInt('5.99', 10); // returns 5
parseInt('7,123', 10); // returns 7
parseInt('20 * 4', 10); // returns 20
Hexadecimal
parseInt('C', 16); // returns 12
parseInt('-0XC', 16); //returns -12
parseInt('EX123', 16); // returns 14
If you were to input an argument that wasn't a number at all into the "string" parameter such as parseInt('helloworld', 10);
you would receive a NaN
response. Furthermore, if you used certain digits that weren't valid based on the numeric representation you define, you would also receive a NaN
response. An example of this would be parseInt('623', 2);
, since 623
is not a valid binary number.
Summary
parseInt()
is an easy way to parse a string value and return a rounded integer. If you're working with a special use-case and require a different numerical base, modify the radix to your choosing. Otherwise, always use 10
as the value for radix as this will guarantee that you won't see any warnings and that your results produce the intended outcomes.