What Is Ajax Programming - Explained
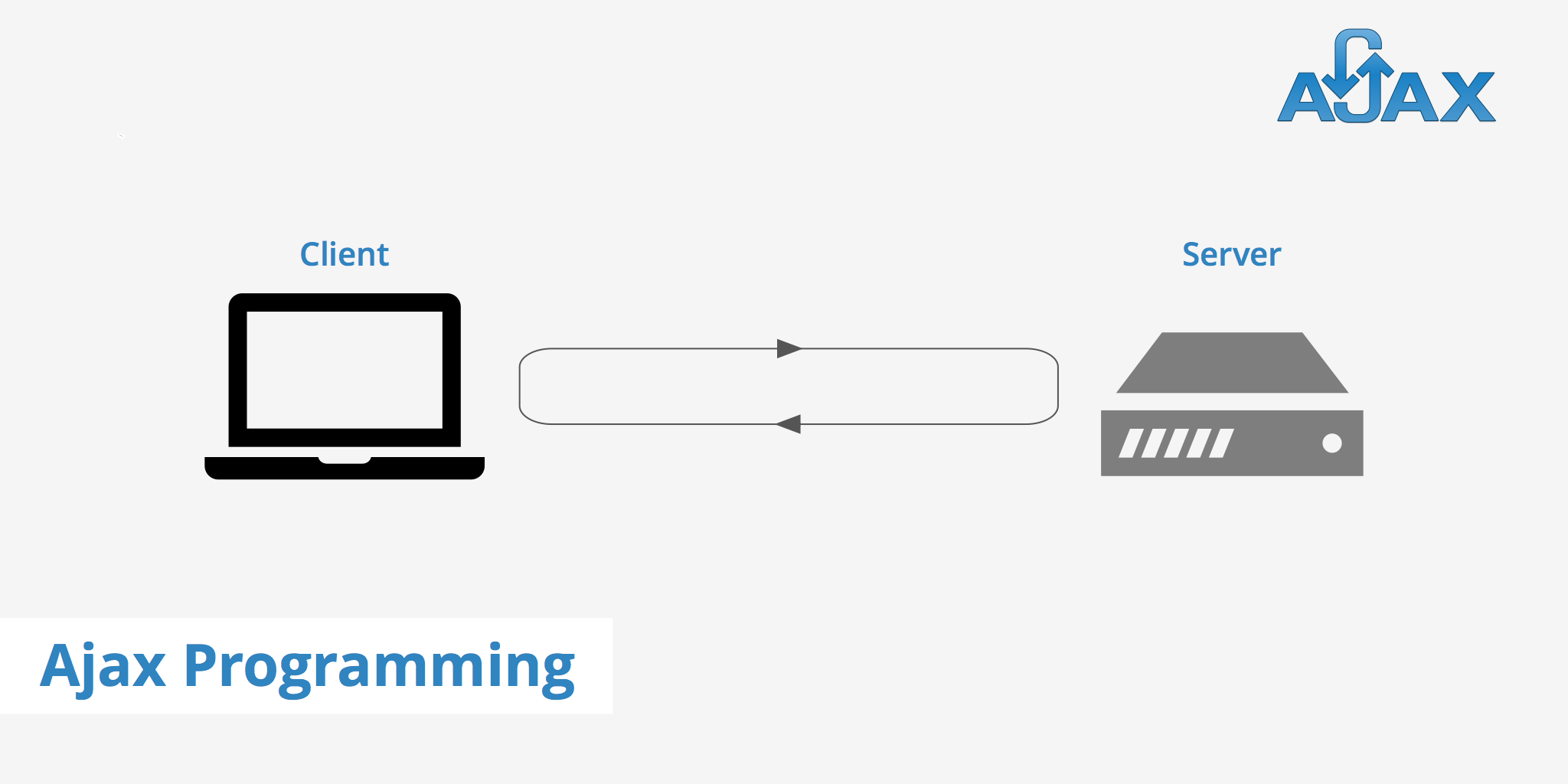
Ajax is short for Asynchronous JavaScript and XML, which refers to a set of web development techniques rather than an actual programming language. Ajax however, is widely used in client side programming (e.g. JavaScript) to allow for data to be sent and received to and from a database / server. What's special about using Ajax programming is that you can exchange data in the background without actually disturbing the user experience. As Wikipedia puts it,
By decoupling the data interchange layer from the presentation layer, Ajax allows for web pages, and by extension web applications, to change content dynamically without the need to reload the entire page.
This method is extremely useful both for website performance and usability. Since asynchronous loading is non-render blocking, it will allow your page's HTML to continue parsing even if it encounters a script tag. From a usability standpoint, visitors can benefit from seeing certain information generated without having to reload the page. This is a huge step forward for improving perceived performance.
How Ajax works
The way Ajax works is quite simple. To help explain, check out the image below which shows a comparison between the conventional method of requesting data from a web server vs using the Ajax method. I'll explain what is taking place on either side in the section below.
Conventional method
Starting from the top of the conventional method, we can see that the browser wants to make a request to the web server for some data. therefore the following takes place.
- An HTTP request is made from the browser to the web server. Therefore, the user must wait for this request to be processed and return a response before they can see the data requested.
- The request reaches the web server and retrieves the appropriate data.
- The requested data is then sent back to the browser and the user is able to see that data.
Ajax method
The following takes place when requesting the same data, however, this time using the Ajax method.
- The browser performs a JavaScript call to the Ajax engine. In other words, create an XMLHttpRequest object.
- In the background, an HTTP request is made to the server and the appropriate data is retrieved.
- HTML, XML, or JavaScript data is returned to the Ajax engine which then delivers the requested data to the browser.
Using this method, the user does not experience any downtime from the point when they made a request to the point when they receive the actual information.
Asynchronous vs synchronous loading
When it comes to loading scripts, traditionally scripts were loading using the synchronous method. This was more or less of a "first-come first-served" process whereas if during the page rendering process, the HTML came across a script tag then it would load that script tag in sequence, thus blocking the parsing of HTML. Once the script was fully loaded, the HTML would continue to parse and load the rest of the web page.
As we can see by the image below, there are 3 synchronous scripts being loaded one after another. **However, there is also an asynchronous script which can be loaded at the same time as the synchronous script which makes for a much more efficient loading process
To learn more about the differences between synchronous and asynchronous loading, check out our Prefer Async Script Loading article
Ajax programming example
As far as loading a script asynchronously goes, this is very easy. To do so, you simply need to add the async attribute to your script tag. For example, if we are loading a script located at js/example.js
then we could define the following.
<script src="example.js" async></script>
As for using Ajax functions, there are a ton of possibilities. First off, a typical Ajax function will have the two following components.
$.ajax(url [, settings])
The Ajax url
parameter defines the URL for which you want to make a request to and the settings
parameter is used to configure the actual Ajax request. Check out the full list of settings options available on the official jQuery and Ajax documentation page.
$.ajax('demo.html', {
success: function(result) {
$('#testdiv').html(result);
}
});
The example above is a simple demonstration of how jQuery and Ajax can be used. The URL for which we make a request to is demo.html
. We then take the result and insert it into the element that contains the ID #testdiv
.
There are many other more complex and in-depth examples out there for using jQuery and Ajax, for more examples check out Sitepoint's How to Use jQuery's $.ajax() Function post.
Summary
As previously mentioned, using Ajax programming methods is not a programming language in and of itself. Rather, it combines multiple technologies in a manner that renders the access of data more efficiently and effectively. Wherever possible, ensure that you are loading your scripts using async and implementing Ajax functions wherever it makes sense so that users can access the information they need much faster.