100+ Awesome Web Development Tools and Resources
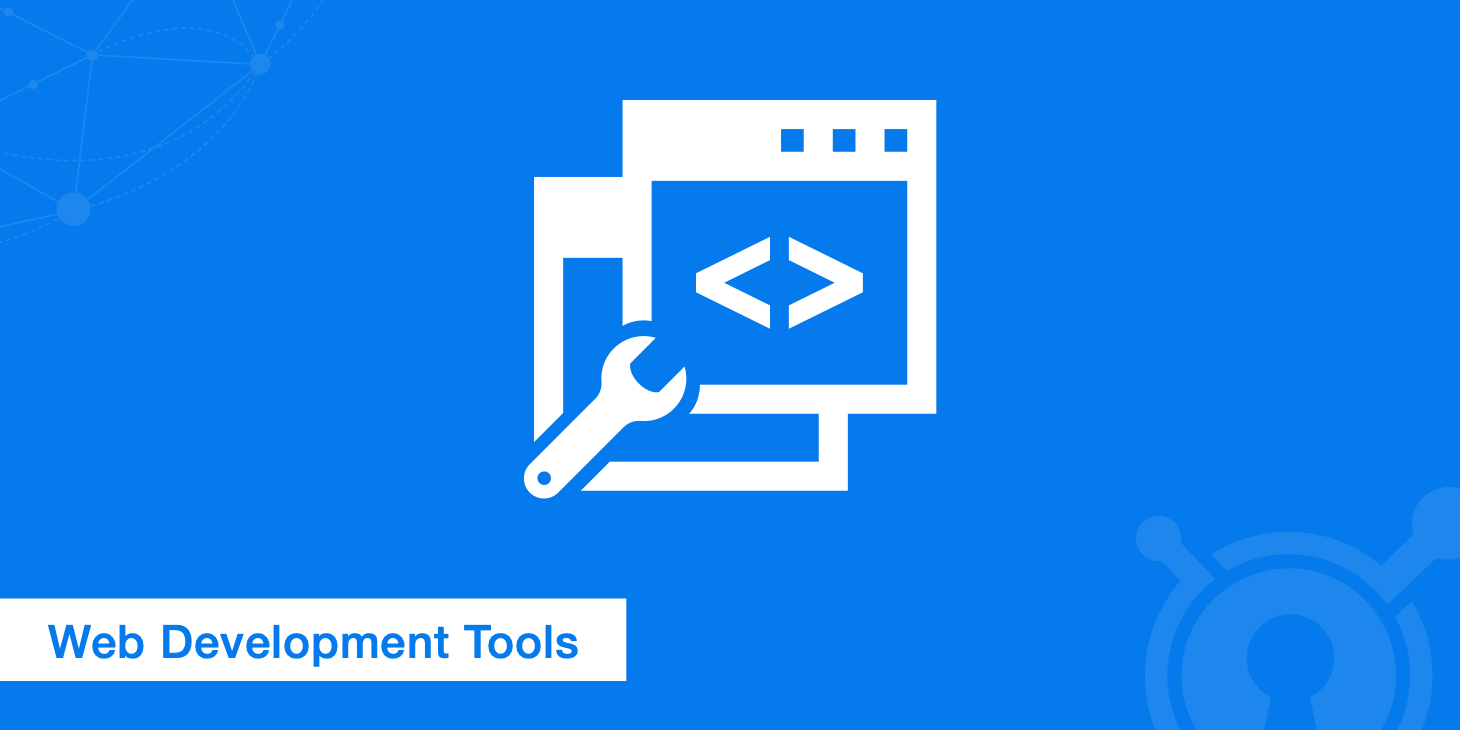
The best and worst thing about being a web developer is that the web is constantly changing. While this is exciting, it also means that web developers must always be proactive in learning new techniques or programming languages, adapting to changes, and be willing and eager to accept new challenges. This could include tasks such as adapting existing frameworks to meet business requirements, testing a website to identify technical problems, or optimizing and scaling a site to perform better with the backend infrastructure. We thought we would compile a comprehensive list of web development tools and resources that can help you be more productive, stay informed, and become a better developer.
Web development tools and resources
A lot of these web development tools below are ones we use at KeyCDN on a daily basis. We can't include everything, but here are a couple of our favorites and other widely used ones. Hopefully, you find a new tool or resource that will aid you in your development workflow. The tools and resources below are listed in no particular order.
JavaScript libraries
JavaScript is one of the most popular programming languages on the web. A JavaScript library is a library of pre-written JavaScript which allows easier access throughout the development of your website or application. For example, you can include a copy of Google's hosted jQuery library by using the following snippet.
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.2.0/jquery.min.js"></script>
- jQuery: A fast, small, and feature-rich JavaScript library.
- BackBoneJS: Give your JS app some backbone with models, views, collections, & events.
- D3.js: A JavaScript library for manipulating documents based on data.
- React: Facebook's JavaScript library developed for building user interfaces.
- jQuery UI: A curated set of user interface interactions, effects, widgets, and themes.
- jQuery Mobile: HTML5-based user interface system designed to make responsive web sites.
- Underscore.js: Functional programming helpers without extending any built-in objects.
- Moment.js: Parse, validate, manipulate, and display dates in JavaScript.
- Lodash: A modern utility library delivering modularity, performance, & extras.
- Vue.js: An open source JavaScript framework used for building user interfaces.
Frontend frameworks
Frontend frameworks usually consist of a package that is made up of other files and folders, such as HTML, CSS, JavasScript, etc. There are also many stand-alone frameworks out there. We are a big fan of Boostrap and the main KeyCDN website is built on it. A solid framework can be an essential tool for frontend developers.
- Bootstrap: HTML, CSS, and JS framework for developing responsive, mobile first projects on the web.
- Foundation: Family of responsive frontend frameworks that make it easy to design beautiful responsive websites, apps and emails that look amazing on any device.
- Semantic UI: Development framework that helps create beautiful, responsive layouts using human-friendly HTML.
- uikit: A lightweight and modular frontend framework for developing fast and powerful web interfaces.
To learn more about the differences between popular frontend framework, check out our list of the top frontend frameworks.
Web application frameworks
A web application framework is a software framework designed to aid and alleviate some of the headache involved in the development of web applications and services.
- Ruby: Ruby on Rails is a web-application framework that includes everything needed to create database-backed web applications, with the MVC pattern.
- AngularJS: Lets you extend HTML vocabulary for your web application. AngularJS is a framework, even though it's much more lightweight and sometimes referred to as a library.
- Ember.js: A framework for creating ambitious web applications.
- Express: Fast and minimalist web framework for Node.js.
- Meteor: Full stack JavaScript app platform that assembles all the pieces you need to build modern web and mobile apps, with a single JavaScript codebase.
- Django: High-level Python Web framework that encourages rapid development and clean, pragmatic design.
- ASP.net: Free, fully supported Web application framework that helps you create standards-based Web solutions.
- Laravel: A free, open source PHP web application framework to build web applications on MVC pattern.
- Zend Framework 2: An open source framework for developing web applications and services using PHP.
- Phalcon: A full stack PHP framework delivered as a C-extension.
- Symfony: A set of reusable PHP components and a web application framework.
- CakePHP: A popular PHP framework that makes building web applications simpler, faster and require less code.
- Flask: A microframework for Python based on Werkzeug and Jinja 2.
- CodeIgniter: Powerful and lightweight PHP framework built for developers who need a simple and elegant toolkit to create full-featured web applications.
Also, make sure to check out KeyCDN's framework integration guides to see how you can implement a CDN with the solutions mentioned above.
Task runners / Package managers
Tasks runners are all about automating your workflow. For example, you can create a task and automate the minification of JavaScript. Then build and combine tasks to speed up development time. Package managers keep track of all the packages you use and make sure they are up to date and the specific version that you need.
- Grunt: JavaScript task runner all about automation.
- Gulp: Keeps things simple and makes complex tasks manageable, while automating and enhancing your workflow.
- npm: Pack manager for JavaScript.
- Bower: A web package manager. Manage components that contain HTML, CSS, JavaScript, fonts or even image files.
- webpack: A module bundler for modern JavaScript applications.
Languages / Platforms
Behind all the web development tools is a language. A programming language is a formal constructed language designed to communicate with a computer and create programs in which you can control the behavior. And yes we realize some of these might not always be referred to as a language.
- PHP: Popular general-purpose scripting language that is especially suited to web development.
- NodeJS: Event-driven I/O server side JavaScript environment based on V8.
- JavaScript: Programming language of HTML and the web.
- HTML5: Markup language, the latest version of HTML and XHTML.
- Python: Programming language that lets you work quickly and integrate systems more effectively.
- Ruby: A dynamic, open source programming language with a focus on simplicity and productivity.
- Scala: Scala is a pure-bred object-oriented language allowing a gradual, easy migration to a more functional style.
- SQL: Stands for structured query language used with relational databases.
- Golang: Open source programming language that makes it easy to build simple, reliable, and efficient software.
- Rust: Systems programming language that runs blazingly fast, prevents segfaults, and guarantees thread safety.
- Elixir: Dynamic, functional language designed for building scalable and maintainable applications.
- TypeScript: Open source programming language that is a superset of JavaScript which compiles to plain JavaScript.
Databases
A database is a collection of information that is stored so that it can be retrieved, managed and updated.
- MySQL: One of the world's most popular open source databases.
- MariaDB: Made by the original developers of MySQL. MariaDB is also becoming very popular as an open source database server.
- MongoDB: Next-generation database that lets you create applications never before possible.
- Redis: An open source, in-memory data structure store, used as a database, cache and message broker.
- PostgreSQL: A powerful, open source object-relational database system.
CSS preprocessors
A CSS preprocessor is basically a scripting language that extends CSS and then compiles it into regular CSS. Make sure to also check out or in-depth post on Sass vs Less.
- Sass: A very mature, stable, and powerful professional grade CSS extension.
- Less: As an extension to CSS that is also backward compatible with CSS. This makes learning Less a breeze, and if in doubt, lets you fall back to vanilla CSS.
- Stylus: A new language, providing an efficient, dynamic, and expressive way to generate CSS. Supporting both an indented syntax and regular CSS style.
If you are just getting started with a CSS preprocessor you might want to make the transition easier by first using a third party compiler, such as the ones below.
Compiler | Sass Language | Less Language | Mac | Windows |
---|---|---|---|---|
CodeKit | ||||
Hammer | ||||
LiveReload | ||||
Prepros | ||||
Scout | ||||
Crunch |
Text editors / Code editors
Whether you're taking notes, coding, or writing markdown, a good text editor is a part of our daily lives!
- Atom: A text editor that's modern, approachable, yet hackable to the core. One of our favorites!
- Sublime Text: A sophisticated text editor for code, markup, and prose with great performance.
- Notepad++: A free source code editor which supports several programming languages running under the MS Windows environment.
- Visual Studio Code: Code editing redefined and optimized for building and debugging modern web and cloud applications.
- TextMate: A code and markup editor for OS X.
- Coda 2: A fast, clean, and powerful text editor for OS X.
- WebStorm: Lightweight yet powerful IDE, perfectly equipped for complex client side development and server side development with Node.js.
- Vim: A highly configurable text editor built to enable efficient text editing.
- Brackets: A lightweight and powerful modern text editor; written in JavaScript, HTML and CSS.
- Emacs: An extensible, customizable text editor with built-in functions to aid in quick modifications of text and code.
- Dreamweaver: Not your typical code editor, however, Dreamweaver can be used to write code and build websites through a visual interface. Learn more in this simple Dreamweaver tutorial.
- SpaceMacs: A text editor design to operate in both Emacs and Vim editor modes.
Markdown editors
Markdown is a markup language in plain text using an easy syntax that can then be converted to HTML on the fly. This is different than a WYSIWYG editor. Markdown editors are sometimes referred to as the in-between WYSIWYG and simply writing code.
- StackEdit: A free online rich markdown editor.
- Dillinger: An online cloud-enabled, HTML5, buzzword-filled Markdown editor.
- Mou: Markdown editor for developers on Mac OS X.
Some of the text editors we mentioned above also support markdown. For example, there is a markdown preview package for atom.
Icons
Almost every web developer, especially frontend developers will at some point or another need icons for their project. Below are some great resources for both free and paid high quality icons.
- Font Awesome: Scalable vector icons that can instantly be customized - size, color, drop shadow, and anything that can be done with the power of CSS.
- IconMonster: A free, high quality, monstrously big and continuously growing source of simple icons. One of our favorites!
- Icons8: An extensive list of highly customizable icons created by a single design team.
- IconFinder: Iconfinder provides beautiful icons to millions of designers and developers.
- Fontello: Tool to build custom fonts with icons.
- Noun Project: Over a million curated icons. Available in both free as well as paid versions for greater customizability.
For more great icon providers, check out our complete list of 16 popular icon library resources.
Git clients / Git services
Git is a source code management system for software and web development known for distributed revision control. When working with teams, using a git client to push code changes from dev to production is a way to maintain the chaos and ensure things are tested so they don't break your live web application or site.
- SourceTree: A free Git & Mercurial client for Windows or Mac. Atlassian also makes a pretty cool team Git client called Bitbucket.
- GitKraken (Beta): A free, intuitive, fast, and beautiful cross-platform Git client.
- Tower 2: Version control with Git - made easy. In a beautiful, efficient, and powerful app.
- GitHub Client: A seamless way to contribute to projects on GitHub and GitHub Enterprise.
- Gogs: A painless self-hosted Git service based on the Go language.
- GitLab: Host your private and public software projects for free.
Web servers
The web server you ultimately end up using will usually depend on a combination of personal preference, functionality, or preexisting infrastructure. Nginx and Apache are the two most widely used web servers around, however, there are other options.
- Nginx: An open source and high-performant web server. Handles static content well and is lightweight.
- Apache: Currently powers almost 50% of all websites. Has a larger community around it and a great selection of modules.
- IIS: An extensible web server created by Microsoft. Offers excellent security and corporate support, therefore is not open source.
- Caddy: A relatively new web server. It is an open source, HTTP/2 web server with automatic HTTPS.
For a complete comparison of today's biggest web servers, check out our Nginx vs Apache article.
API tools
Web developers typically deal with APIs on a daily basis. They are essential in today's web development environment, however, can sometimes be difficult to deal with in terms of monitoring, creating, or combining. Thankfully, there are a variety of tools available to make working with APIs much more efficient.
- Runscope: An API performance testing, monitoring, and debugging solution.
- Zapier: Connect the APIs of various apps and services in order to automate workflows and enable automation.
- Postman: Complete API development environment. Everything from designing, testing, monitoring, and publishing.
- SoapUI: Advanced REST and SOAP testing tool. Ability to perform functional testing, security testing, performance testing, etc.
Local dev environments
Depending upon what OS you are running or the computer you currently have access to, it might be necessary to launch a quick local dev environment. There are a lot of free utilities that bundle Apache, mySQL, phpmyAdmin, etc. all together. This can be a quick way to test something on your local machine. A lot of them even have portable versions.
- XAMPP: Completely free, easy to install Apache distribution containing MariaDB, PHP, and Perl.
- MAMP: Local server environment in a matter of seconds on OS X or Windows.
- WampServer: Windows web development environment. It allows you to create web applications with Apache2, PHP and a MySQL database.
- Vagrant: Create and configure lightweight, reproducible, and portable development environments.
- Laragon: A great fast and easy way to create an isolated dev environment on Windows. Includes Mysql, PHP Memcached, Redis, Apache, and awesome for working with your Laravel projects.
Diff checkers
Diff checkers can help you compare differences between files and then merge the changes. A lot of this can be done from CLI, but sometimes it can be helpful to see a more visual representation.
- Diffchecker: Online diff tool to compare text differences between two text files. Great if you are on the go and quickly need to compare something.
- Beyond Compare: A program to compare files and folders using simple, powerful commands that focus on the differences you're interested in and ignore those you're not.
A lot of the free text editors we mentioned above also have plugins or extensions which allow you to diff or compare your files.
Code sharing / Code experimenting
There is always that time when you are on Skype or Google hangout with another developer and you want him or her to take a quick look at your code. There are great team tools for sharing code like Slack, but if they aren't a member of your team there are some great quick alternatives. Remember not to share anything secure.
- JS Bin: Tool for experimenting with web languages. In particular HTML, CSS and JavaScript, Markdown, Jade and Sass.
- JSfiddle: Custom environment to test your JavaScript, HTML, and CSS code right inside your browser.
- codeshare: Share code in real time with other developers.
- Dabblet: Interactive playground for quickly testing snippets of CSS and HTML code.
Collaboration tools
Every great development team needs a way to stay in touch, collaborate, and be productive. A lot of teams work remotely now. The team at KeyCDN is actually spread across many different continents. Tools like these below can help employees streamline their development workflow.
- Slack: Messaging app for teams that is on a mission to make your working life simpler, more pleasant, and more productive.
- Trello: Flexible and visual way to organize anything with anyone. One of our favorites, we use this at KeyCDN!
- Glip: Real-time messaging with integrated task management, video conferencing, shared calendars and more.
- Asana: Team collaboration tool for teams to track their work and results.
- Jira: Built for every member of your software team to plan, track, and release great software or web applications.
Inspiration
We all need inspiration at some point or another. For frontend developers especially, from time to time, it can be beneficial to check out what other people are doing. This can be a great source of inspiration, new ideas, and making sure your web application or site doesn't fall behind the times.
- CodePen: Show off your latest creation and get feedback. Build a test case for that pesky bug. Find example design patterns and inspiration for your projects.
- Dribble: A community of designers sharing screenshots of their work, process, and projects.
- Behance: Another community-driven resource where users can showcase and discover creative work.
Website speed test tools
The speed of a website can be a critical factor to its success. Faster loading websites can benefit from higher SEO rankings, higher conversion rates, lower bounce rates, and a better overall user experience and engagement. It is important to take advantage of the many free tools available for testing website speed.
- Website Speed Test: A page speed test developed by KeyCDN that includes a waterfall breakdown and the website preview.
- Google PageSpeed Insights: PageSpeed Insights analyzes the content of a web page, then generates suggestions to make that page faster.
- Google Chrome DevTools: Set of web authoring and debugging tools built into Google Chrome.
- Dotcom-Tools Speed Test: Test the speed of your website in real browsers from 25 locations worldwide.
- WebPageTest: Run a free website speed test from multiple locations around the globe using real browsers (IE and Chrome) and at real consumer connection speeds.
- Pingdom: Test the load time of that page, analyze it and find bottlenecks.
- GTmetrix: Gives you insight on how well your site loads and provides actionable recommendations on how to optimize it.
You can see a more in-depth list on our post about website speed test tools.
Web development communities
Every web developer has been there. They have a problem and what do they do? Well, they go to Google to find a quick answer. The web offers so much content right at our fingertips that it makes it easy to diagnose and troubleshoot problems when they arise. Check out a few good web development communities below.
- Stack Overflow: Community of 4.7 million programmers, just like you, helping each other.
- Frontend Front: A place where frontend developers can ask questions, share interesting links, and show their work to the rest of the community.
- Hashnode: Global community for software developers to connect and learn programming from each other.
- Refind: Community of founders, hackers, and designers who collect and share the best links on the web.
- Facebook WordPress Front End Developers Group: WordPress Front End Developers is a group for devs to ask questions, share their work, discuss emerging trends, and collaborate.
- LinkedIn Web Design and Development Professionals Group: Networking and information sharing resource for professional Web Designers, Web Developers and Web Masters.
- LinkedIn Web Site Development Group: Website design & programming.
- LinkedIn PHP Developer Group: PHP, Mysql, Drupal, Joomla, Zend, Cake, MVC.
- Webdeveloper.com: Where web developers and designers learn how to build web sites, program in HTML, Java and JavaScript.
- Sitepoint Forums: Web development discussion.
- /r/perfmatters: The #1 subreddit about web performance and web development.
- /r/webdev: What's new for web developers.
Web development newsletters
- wdrl.info: A handcrafted, carefully selected list of web development related resources. Curated and published usually every week.
- webopsweekly.com: A weekly newsletter for Web developers focusing on web operations, infrastructure, deployment of apps, performance, and tooling, from the browser down to the metal.
- webtoolsweekly.com: A frontend development and web design newsletter with a focus on tools. Each issue features a brief tip or tutorial, followed by a weekly round-up of various apps, scripts, plugins, and other resources.
- smashingmagazine.com: Smashing Magazine is an online magazine for professional web designers and developers. Useful tips and valuable resources sent out every second Tuesday.
- fridayfrontend.com: Frontend development links tweeted daily, emailed weekly.
- /dev tips: Receive a developer tip, in the form of a gif, in your inbox each week.
And of course, you can subscribe to our newsletter if you haven't already on the right-hand side of this post.
Summary
As you can see there are hundreds of web development tools and resources available to help streamline your development workflow and hopefully aid you in being more productive. Again we can't list every tool or resource, but if we forgot something important, feel free to let us know below in the comments.