5 Part PHP Cheat Sheet
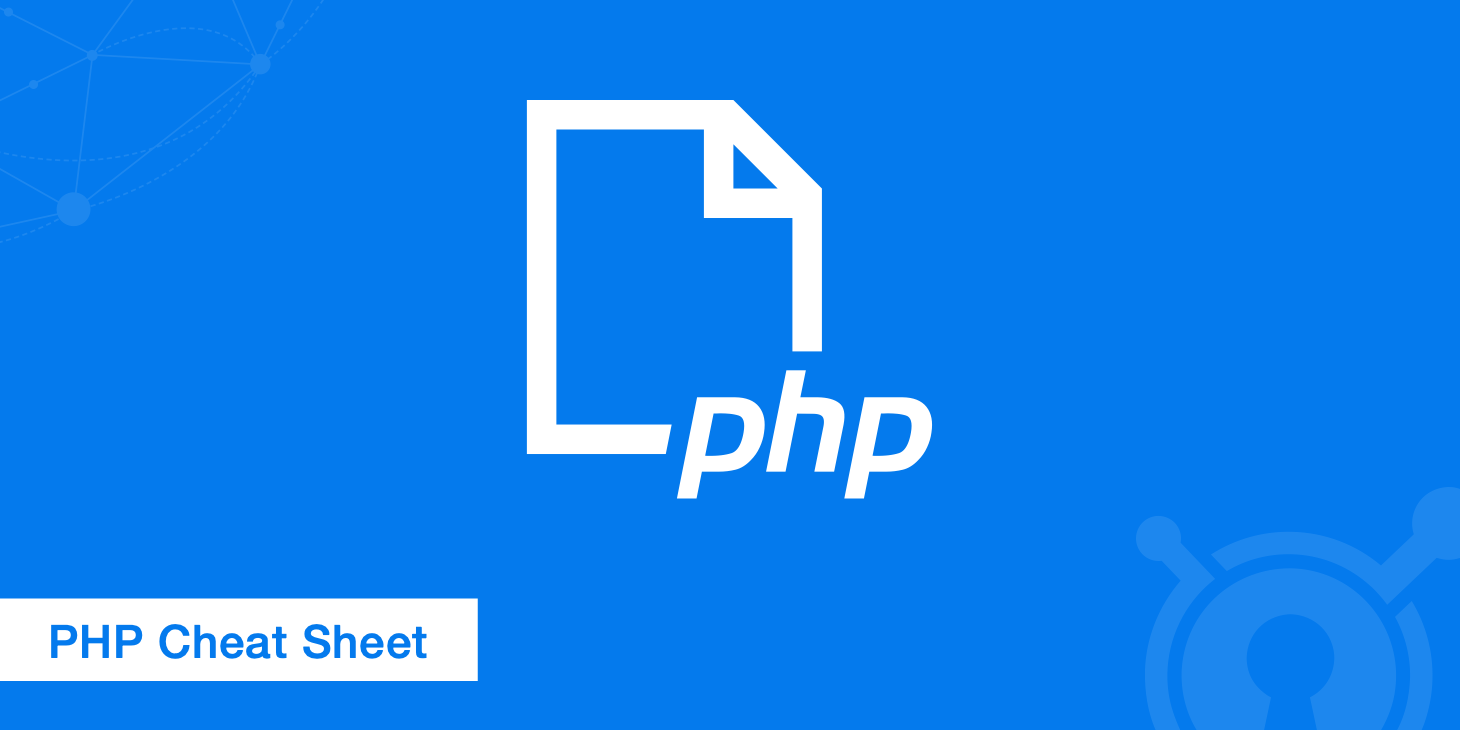
PHP is perhaps the most popular general purpose scripting language for web developers, but its capabilities extend well beyond building websites. Of course, immense capabilities often come with a steep learning curve. Fortunately, PHP is straightforward enough for coding novices yet powerful enough for professional programmers.
Its massive list of features may seem intimidating at first; however, anyone with even very little knowledge of coding should be able to write simple PHP scripts. That said, no one can expect to become an expert overnight, so this PHP cheat sheet can help beginners and experts out in a pinch.
1. PHP basics
In case you need a quick crash course, this section will cover the very basics of PHP.
Unlike client side JavaScript, PHP code is executed on the server, which generates HTML to send to the client. Therefore, the client sees the results of the script but not the underlying code. It is possible to configure your server to process all HTML files with PHP so that users have no way of knowing what lies beneath.
PHP code is embedded into HTML and must be set off by the start and end processing instructions, <?php
and ?>
, as illustrated in the example below. The following code would output PHP goes here!
:
<!DOCTYPE html>
<html>
<head>
<title>First Example</title>
</head>
<body>
<?php
echo "PHP goes here!";
?>
</body>
</html>
The echo statement allows you to output strings, which are written within quotation marks and terminated with a semicolon.
According to W3Techs, more than 75% of web servers deploy PHP. However, because PHP is an open source language that has evolved naturally rather than being deliberately engineered, it's easy to design applications with security vulnerabilities. OWASP has a fantastic PHP security cheat sheet to ensure that your PHP-based application remains secure.
2. PHP modes
PHP scripts execute in multiple modes including the Apache module, CGI, and FastCGI. If you're using PHP for a project, this section can help you figure out where to start. There are alternatives, but these are the main ones:
I. mod_php (Apache module)
Using mod_php embeds the PHP interpreter in each Apache process when it spawns. Therefore, no external processes are need because Apache workers execute PHP scripts themselves. This comes in handy for websites that receive requests heavy with PHP code like WordPress, Drupal, or Joomla. The interpreter caches information so that the same tasks don't have to be repeated every time a script executes. The trade off is that Apache processes leave a bigger footprint as they eat up more memory.
mod_php benefits
- No external processes needed
- High performance for websites with a lot of PHP
- Settings may be configured and customized within .htaccess directives
mod_php drawbacks
- PHP interpreter gets loaded even with non-PHP content
- Since files containing PHP scripts are often owned by the web server, you can't always edit them through FTP later on
II. CGI
Using a CGI application to execute PHP scripts is considered archaic, but it is still practical for a few purposes. The biggest benefit to using CGI is that code execution is kept separate from your server. Since the PHP interpreter only gets called when needed, static content remains secure on the server side; however, because a new process must be created wherever PHP code is used, this mode can get very resource intensive very fast. Therefore, CGI is not recommended for projects that rely heavily on PHP.
CGI benefits
- More secure than mod_php
CGI drawbacks
- Outdated performance
III. FastCGI
FastCGI offers a compromise between the security of CGI and the performance of mod_php. Scripts are executed by the interpreter outside of the server, and each request passes from the server to FastCGI through a network socket. The server and the PHP interpreter may then be split into separate environments, which improves scalability. The same goal can also be accomplished by using nginx in conjunction with mod_php so that nginx takes care of most non-dynamic requests.
The major limitation of running PHP with FastCGI is the inability to use PHP directives that have been defined in a .htaccess file, which many popular scripts require. If you are using a Plesk Panel server, you can work around this issue by setting PHP directives on a per domain basis using a custom php.ini
file.
FastCGI benefits
- Offers greater security than mod_php
- Static content isn't processed by the PHP interpreter
- Files may be managed by FTP users without the need for changing permissions afterwards
FastCGI drawbacks
- PHP directives defined in .htaccess files cannot be used without a workaround
- PHP requests are passed from the server
If you're working on a smaller project, feel free to use whichever mode you prefer. FastCGI is best suited for running CMS applications like WordPress while mod_php might be better if you rely on directives in .htaccess files, so long as you take extra security precautions.
3. PHP functions
Functions stand in for commonly used chunks of code so that you don't have to keep copying and pasting the same snippets over and over again. It would be impossible to comprehensively cover the long list of PHP functions in one article. Indeed, you can likely find a PHP cheat sheet geared toward any specific purpose you need. This section will provide in-depth explanations of commonly used functions.
I. String manipulation
strlen()
The strlen()
function passes a string or variable and returns the number of characters including spaces. For example:
<?php
$name = "Luke Perry";
echo strlen($name); // 10
?>
substr($string, $start, $length)
The substr()
function returns a specified part of a string. There are three parameters that you can pass along.
- The first parameter,
$string
, is the name of the string or the variable containing the string. - The second parameter,
$start
, designates which character you want to start from; entering 0 will indicate to start from the first character, 1 will start from the second character and so on. Entering a negative value will start counting backward from the end of the string. - The third and final parameter,
$length
, indicates how many characters to return after$start
. If a$length
parameter isn't provided, the function returns the entire remainder of the string. When$length
is less than or the same as the$start
value, it simply returns false.
Therefore, the function works as follows:
<?php
$name = "Luke Perry";
echo substr($name, 0, 3); // Luk
echo substr($name, 1); // uke Perry
echo substr($name, -4, 3); // err
?>
strtoupper()
The strtoupper()
function converts strings to all uppercase while strtolower()
converts strings to all lowercase, which comes in handy for case sensitive operations. For example:
<?php
$name = "Luke";
echo strtoupper($name); // LUKE
echo strtolower($name); // luke
?>
strpos($haystack,$needle,$offset)
The strpos()
function helps you determine the location of a substring within a larger string. There are three parameters.
- The first,
$haystack
, is the main string you wish to search. - The second,
$needle
, is the specific cluster of characters you are seeking. These parameters alone will return the position of$needle
expressed as a number. - The third parameter,
$offset
, is optional. It must be a number indicating where to start the search from, so the value cannot be negative.
Notice how in the following example the last line returns false because the function is case sensitive:
<?php
$name = "Luke Perry";
echo strpos($name, "L"); // 0
echo strpos($name, "e Perry"); // 3
echo strpos($name, "p"); // false
?>
The strpos()
function can be used along with if statements as demonstrated below:
<?php
$string = "I am a string.";
$search = "PHP";
if (strpos($string, $search) === false) {
echo "Sorry! '$search' cannot be found in '$string'.";
}
?>
The above would echo Sorry! 'PHP' cannot be found in 'I am a string.'
.
II. Arithmetic functions
round($val, $precision, $mode)
The round()
function, as its name implies, rounds numbers that contain decimal points. You may round up or down to a whole number or a specified decimal place. Once again, there are three parameters.
- The first one,
$val
, is the value that you want to round. - The next one,
$precision
, is optional as it indicates the number of decimal places to round to. - The final one,
$mode
, indicates the type of rounding.
There are four options: PHP_ROUND_HALF_UP
, PHP_ROUND_HALF_DOWN
, PHP_ROUND_HALF_EVEN
, and PHP_ROUND_HALF_ODD
. You can find more information about rounding and other functions in the PHP manual.
Two other math functions that have to do with rounding, ceil()
and floor()
, round up and down respectively. They provide an easier way to round to the nearest integer. For example:
<?php
$number = 7.55686878;
echo
ceil($number); // 8
floor($number); // 7
?>
III. Random number generation
rand($min, $max)
The rand()
function returns a random number between two specified numbers. Its two parameters are both optional. The first, $min
, sets the lowest value while the second, $max
, sets the maximum. If no values are provided, $min
defaults to 0 and $max
returns getrandmax()
. For example:
<?php
echo rand(); // 15748
echo rand(); // 490
echo rand(7, 9); //7
?>
For some reason, some Windows systems set getrandmax()
at 32767, but you can designate a larger $max
value.
IV. Fun with array functions
The array()
function obviously lets you store multiple values in one single variable, but there are plenty of additional array functions that you should keep in mind.
array_push($array, $value1, $value2)
The array_push()
function adds new elements to the end of an array. You must choose the array and at least one value. There is no limit to how many values you can push.
<?php
$states = array();
$array = array_push($states, "Montana");
$array = array_push($states, "North Carolina");
$array = array_push($states, "California");
echo $array; // returns 3
var_dump($states);
?>
An even easier way to do this is by listing each element in a single call. For example:
<?php
$states = array(); // target array
$array = array_push($states,
"Montana",
"North Carolina",
"California");
echo $array; // returns 3
var_dump($games);
?>
The two previous examples produce the same outcome. Now, if you echo array_push()
, it returns the number of variables to be pushed into the array. If you then use the var_dump()
function, it will return the following:
array(3) {
[0] =>
string(7) "Montana"
[1] =>
string(14) "North Carolina"
[2] =>
string(10) "California"
}
sort($array, $sort_flags)
sort()
is another array function that does exactly what you think it does. After designating the array to be sorted, you have the option to set filters by entering a value for $sort_flags
. The developer's manual has its own cheat sheet for $sort_flags
. The function sorts alphabetically or numerically by default. For example:
<?php
$states = array(
"Montana",
"North Carolina",
"California");
sort($states); // array to sort
echo join(", ", $states);
//output - California, North Carolina, Montana
?>
join(glue, array)
or implode(glue, array)
You must use the join()
function to echo sorted arrays. The implode()
function allows you to do the exact same thing, so the same rules reply to both functions.
The first parameter, glue, is actually optional; it indicates what to put between the variables. In the above example, glue is a comma. The second parameter is obviously the array you wish to sort.
rsort($array, $sort_flags)
The rsort()
function does the same thing as sort()
but in reverse. For example:
<?php
$states = array(
"Montana",
"North Carolina",
"California");
rsort($states); // array to sort
echo join(", ", $states);
//output - Montana, North Carolina, California
?>
V. Advanced PHP functions
For information about more advanced PHP functions, Envato has a useful list of some lesser known capabilities of PHP.
4. PHP commands
PHP has all of the commands you'd expect in a scripting language, which are too numerous to cover in-depth here. Fortunately, 1Keydata has an excellent tutorial on PHP commands in addition to other information about how to use PHP to its full potential.
5. More PHP cheat sheet resources
You can find dozens of PHP cheat sheets for all types of specific purposes floating around online. Here are a couple notable charts:
If you don't use PHP for all of your projects, it's easy to forget some of the ins-and-outs of the language. This article aimed to provide a quick reference for the most frequently used PHP functions and features. Feel free to come back whenever you need a refresher.