Comprehensive Node Version Manager (NVM) Tutorial
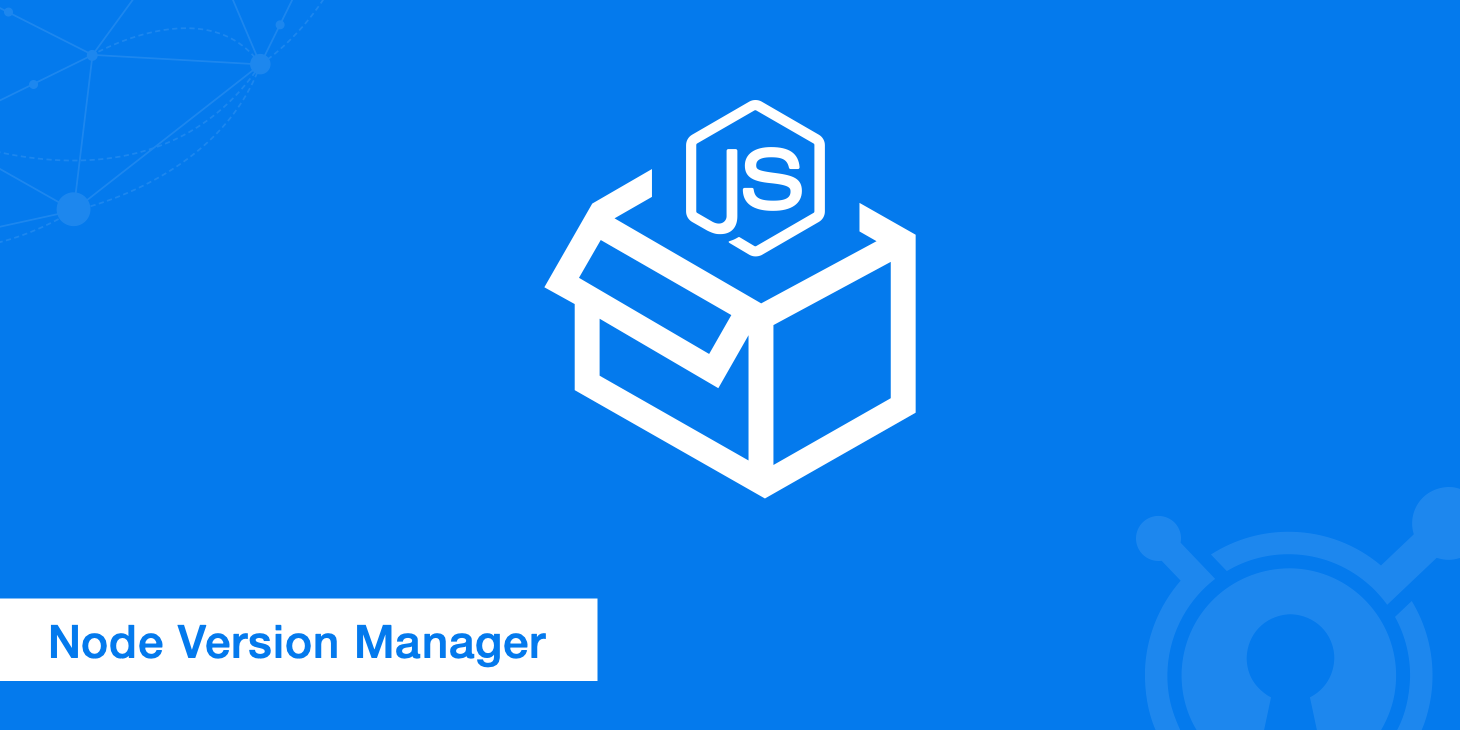
If you build Node.js applications, you may want to use different versions of Node. Fortunately, there is an easy way to install and manage them all from a single machine thanks to Node Version Manager. This guide covers all of the commands you need to know to start switching Node versions with no hassle.
What is Node.js?
Node.js is an open source JavaScript run-time environment used for making web servers and other networking tools. Its core functionality is supported by modules based on an API intentionally designed to make writing server applications less complex. Node.js applications run on Linux, macOS, Windows, NonStop and Unix servers. They can be written with any language that can compile to JavaScript such as CoffeeScript, Dart and TypeScript.
Node.js is similar to PHP, but there are some notable differences. In PHP, functions tend to block until completion, which means that commands execute one at a time. In, Node.js, commands are executed in parallel, and callbacks are used to determine completion or failure.
Node.js version updates
A new release of Node.js is cut from the GitHub master branch every six months around April and October. Whenever a new odd-numbered version comes out, the most recent even version goes to long-term support, or LTS. Versions under LTS receive active support for 18 months followed by an additional 12 months of maintenance support. Meanwhile, active versions get non-breaking backports of any changes implemented in the most current version a few weeks after release. Maintenance releases periodically receive critical fixes and documentation updates.
The Technical Steering Committee of the Node.js Foundation oversees LTS release policies and strategies while the LTS Working Group actually manages support.
What is Node Version Manager (NVM)?
Node Version Manager is a tool that allows programmers to seamlessly switch between different versions of Node. You can install each version with a single command and set a default via the command line interface.
Which OS supports Node Version Manager?
NVM was originally designed for Linux and OS X, but there is a similar program called NVM Windows. While these programs have some slight differences, the basic commands for installing, listing and switching between Node.js versions are identical except for where noted.
Installing NVM for OS X and Linux
First, make sure the tool is compatible with the specific version of the OS you're running. You don't technically have to remove your existing node installations, but it's still a good idea to do so anyway. While you're at it, consider removing any npm installations you have since they could cause issues.
A C++ compiler is also required to support versions of Node.js prior to 0.8.6. Even if you typically only work with LTS or modern releases, you might as well install a C++ compiler if you don't have one. If you're using OS X, your best option is Xcode. To install it, run this command:
xcode-select --install
If you're using Linux, run the following commands to install the build-essential package with the Advanced Package Tool:
sudo apt-get update
sudo apt-get install build-essential
Finally, you can install Node Version Manager using either curl or Wget. For curl, run the following from your terminal:
curl -o- https://raw.githubusercontent.com/creationix/nvm/v0.33.5/install.sh | bash
To use Wget, run this command:
wget -qO- https://raw.githubusercontent.com/creationix/nvm/v0.33.5/install.sh | bash
Before you install, make sure to check for the latest available NVM version. These examples assume you're installing v0.33.5. The above commands will clone the repository to ~/.nvm
and apply changes to your bash profile so that you can access Node Version Manager from anywhere in your terminal.
Installing NVM for Windows
Before you get started, you should uninstall any existing version of Node.js from your system to prevent potential problems during installation. Next, head over to GitHub and download nvm-setup.zip. After you open it, just follow the on-screen instructions to complete setup.
The CLI may throw the following error after installation:
Fortunately, there is an easy fix:
- Navigate to the
C:\Users\{username}\AppData\Roaming\nvm
directory. - Copy
settings.txt
. - Paste it to
C:\
. - Run the setup file again.
How to use Node Version Manager
There are a few important things to be aware of in order to be able to use Node Version Manager effectively and efficiently. The sections below discuss these topics and will show examples on how to accomplish certain tasks.
Installing different versions of Node.js
The version manager makes it extremely easy to install multiple versions of Node.js with a single command. Just run the install command followed by the desired version. For example, if you wanted to install Node.js version v6.5.0, you'd run this command:
nvm install v6.5.0
Since the tool follows SemVer, you can install patches by simply running the install command and the patch number. To get a complete list of available versions for Linux and OS X, run:
nvm ls-remote
If you're using a Windows version, use this command instead:
nvm ls available
npm
If you ever need to uninstall an instance of Node, just run nvm uninstall followed by the version number.
Global npm packages
It should be noted that globally installed npm packages aren't shared between various version of Node.js because they could cause incompatibility issues. Therefore, Node Version Manager will simultaneously install a compatible version of npm every time you install a version of Node.js. Since each Node instance could come with its own npm version, you may want to run npm -v
to check which one is currently in use. On the upside, users needn't worry about sudo privileges when installing global packages. If you want to reinstall npm global packages for a specific version of Node.js while also installing a new version, you can do so as follows:
nvm install v6.5.0 --reinstall-packages-from=4.2
The above command installs version 6.5.0 and its corresponding npm version before reinstalling any npm packages you had previously installed for version 4.2.
Simply supplying the version number will always target Node.js, but you can also install io.js instances by adding the iojs-v
prefix. For example, to install io.js version 3.2.0, run:
nvm install iojs-v3.2.0
Aliases
To make things even easier, Node Version Manager lets you use aliases to target versions without using a specific version number. These aliases include:
- node: installs the most recent stable version of Node.js
- unstable: installs the most recent unstable version of Node.js
- iojs: installs the most recent stable version of io.js
Therefore, if you just wanted the latest version of Node.js, you could run:
nvm install node
There is also a default alias. To designate the latest version of Node.js as your default, run:
nvm alias default node
You can even make your own aliases using the following format:
nvm alias my-favorite 6.5.0
The above command creates an alias called my-favorite
for version 6.5.0. In the future, you can switch to 6.5.0 as follows:
nvm use my-favorite
If you ever want to ever get rid of this alias, just run:
nvm unalias my-favorite
Switching between Node.js versions
Whenever you install a new version of Node.js, it will automatically be put to use. To switch between versions, we use the command nvm use, which works basically the same as the install command. Just add a version number or an alias. For example, if you wanted to switch to the latest version of Node.js, you'd simply run:
nvm use node
To get a list of the Node.js versions you have installed as well as your assigned aliases, just run:
nvm ls
The version that's currently in use will appear in green and have an arrow pointing to it while the other versions will appear blue. Alternatively, you can use the following command to check which version you're currently using:
nvm current
Additional commands
There are a few other commands that you may occasionally want to use. To run a command for an installed version without switching node variables, use the following format:
nvm run 6.5.0 --version
To target a specific version while running a command on a sub-shell, use this format:
nvm exec 6.5.0 node --version
If you want to view the path to the Node.js executable of a specific version, use this format:
nvm which 6.5.0
You can also designate which Node.js version you want to use for specific projects by creating
.nvmrc
files in project directories. There is also a plugin called avn that lets you deeply integrate into your shell by automatically invoking Version Manager whenever you change directories.
Troubleshooting
If you attempt to install a Node version, and the installation fails, you should delete the Node downloads from src. Otherwise, you could receive an error when you try again.
If you're having trouble with installations because there are incompatibilities between your system and the official binary packages, try using the
-s
option to force install from the source. For example, if you wanted to install version 6.5.0, you'd run:nvm install -s 6.5.0
If you're running OS X, and the Version Manager command line isn't available after you've set everything up, you could be missing a
.bash_profile
file. To remedy this problem, try running the following command before you re-run the installer script:touch ~/.bash_profile
If you're still having issues, try opening up the existing
.bash_profile
file in your text editor of choice and add this line:source ~/.bashrc
The benefits of Node Version Manager
Aside from saving time and effort, being able to switch between Node versions has a few significant benefits. For instance, let's say a tool claims to support just one specific version of Node.js, but you want to see if it works with another version that you prefer. If you encounter bugs, Version Manager makes it simple to switch node versions for quick troubleshooting. Otherwise, you'd have to continuously uninstall and reinstall node versions and their global packages to switch back and forth.
If you're using a tool that's still in the developer preview phase, Node Version Manager gives you a way to get around the hurdles that can come with each update. For example, when SharePoint Framework was is the preview phase, each drop came with a new version of the Yeoman generator. Therefore, you could be in a situation where you're able to work locally with the latest developer preview, but you'd have to either wait days or even weeks for the supported libraries to become available in Office 365, or you'd just have to hold off installation until the libraries arrived. With Node Version Manager, you can easily keep two versions going with the old and new generators.
If you attend an event like DevKitchen or a workshop where you're given some tools in a pre-release state, Version Manager makes it easy to store and save them for later. Instead of using your main Node.js install, you can quickly switch to a different version of Node.js, work in that environment and save it for later. That way, you will have easy access to those tools later on even if they are unavailable elsewhere.
Summary
Knowing how to use Node Version Manager can help you save a lot of time, which is usually extremely important to development teams. Keep this guide handy just in case you ever need a refresher, but with such a slight learning curve, you'll likely memorize the commands fairly quickly