How to Seamlessly Integrate Brotli Compression with webpack
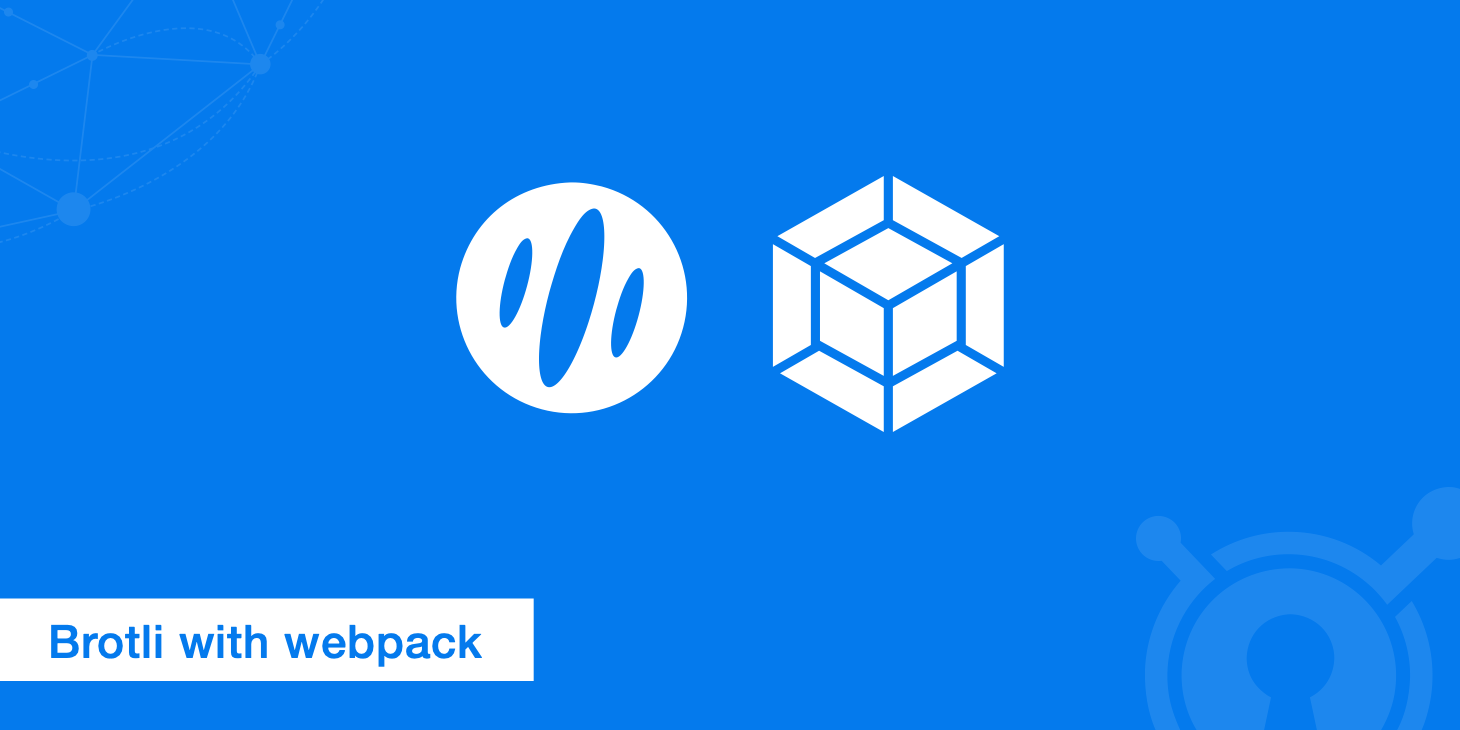
When talking about web performance it's always interesting to hear about new ways to make the process simpler and more efficient. Brotli and webpack are two technologies that have a proven track record of achieving both of these things.
That's why in this article, we're going to explore how developers can use Brotli with webpack in order to further simplify the process of reducing the size of compressible assets.
What is webpack?
webpack is module bundler. What this means is that it takes your modules with dependencies and bundles them into static assets which represent those modules with dependencies.
With webpack everything can be a module. This includes JS, HTML, CSS, and images. All of which (minus images) are compressible with algorithms such as Gzip or Brotli.
The creators of webpack developed their module bundler with the following goals in mind:
- To offer unparalleled customization
- To reduce initial load times
- To divide dependency trees into chunks that are loaded on demand
- To allow every static asset to be a module
- To allow integration of third party libraries as modules
webpack is a highly extensible tool and has been known to run its build process faster than similar module bundlers. Apart from these features, webpack also has a massive and growing community around it. With over 37,000 stars on GitHub at the time of writing this article, and tons of community and corporate sponsors, webpack continues to make module bundling an easier task for everyone.
Read our complete guide on webpack to learn more.
What is Brotli?
Brotli is still a relatively new compression algorithm developed by Google. It provides better compression results than the current standard (Gzip) and test results have shown a 20-26% higher compression ratio when comparing Brotli to Zopfli (another modern compression algorithm).
Brotli support continues to grow and at the time of writing this article, most browsers (including all major browsers) support Brotli compression.
Therefore, if you're offering Brotli-compressed versions of your assets to visitors, they'll be able to access them in less time due to their smaller file sizes. There are various tests which show how much smaller files compressed with Brotli are compared to other compression algorithms, however, the following chart from Sam Saffron gives a nice overview of the difference in file sizes between varying algorithms and quality levels.
To learn more about this new compression algorithm, check out our Brotli compression guide.
How to use Brotli with webpack
First off, you'll need to ensure that your origin server supports Brotli (you can use our Brotli Test tool to verify if it does). This is required since otherwise, your origin server will not be aware that it should deliver Brotli-compressed assets to compatible browsers when available. If you're using Nginx, install the ngx_brotli
module and set the brotli_static
directive to on
. This tells the server to check for pre-compressed Brotli assets (which is what we'll create in the following section).
Setting up webpack to compress your bundled assets with Brotli is a pretty simple process. For the purposes of this example, we've set up a fresh installation of webpack and configured our files to resemble what is outlined in webpack's getting started documentation. This gave us a src
directory with an index.js file
, a dist
directory with an index.html
file and a webpack.config.js
file.
Once this was configured, the brotli gzip plugin for webpack plugin was installed using the following command:
npm install --save-dev brotli-gzip-webpack-plugin
The following snippet was then added to our webpack.config.js
file.
var BrotliGzipPlugin = require('brotli-gzip-webpack-plugin');
module.exports = {
plugins: [
new BrotliGzipPlugin({
asset: '[path].br[query]',
algorithm: 'brotli',
test: /\.(js|css|html|svg)$/,
threshold: 10240,
minRatio: 0.8
quality: 11
}),
new BrotliGzipPlugin({
asset: '[path].gz[query]',
algorithm: 'gzip',
test: /\.(js|css|html|svg)$/,
threshold: 10240,
minRatio: 0.8
})
]
}
Custom arguments such as quality
(as seen in the snippet above) can be added to specify the Brotli compression level of your assets. The default quality level is 11
, which is the highest setting, however can be reduced if desired.
Now we can run the build process with npx webpack --config webpack.config.js
or npm run build
(if you've set up the npm shortcut script). Once complete, webpack will create an uncompressed bundle.js
file, a bundle.js.br
file, and a bundle.js.gz
file.
As can be seen in the screenshot above, the Brotli-compressed version of bundle.js
is the smallest in file size. It's 7.2 times smaller than the uncompressed version and is 22.6% smaller than the Gzipped version.
Of course, generating a Gzipped-compressed version of assets is also important as not all browsers currently support Brotli. Therefore unsupported browsers will request Gzip-compressed assets instead of Brotli.
Benefits of using Brotli with webpack
Using either Brotli or webpack alone comes with various benefits. However using them together allows you to streamline the process of bundling assets while simultaneously optimizing them for faster delivery.
For example, if you were to simply use the ngx_brotli
module to compress Brotli assets on the fly, this would use server resources each time a request is made for a compressible asset. Therefore, not only are server resources unnecessarily being used, but it also takes time to compress these assets each time.
By pre-compressing your Brotli assets with webpack, your origin server doesn't need to use additional resources to compress the files each time a request is made as they're already created and stored on the origin server. Therefore, the ngx_brotli
module, just needs to find them and deliver them.
Furthermore, since KeyCDN supports Brotli, you can easily cache Brotli-compressed assets on our network of global edge servers. Simply ensure that your origin server is configured to support Brotli, enable the Cache Brotli feature in your Zone, and the CDN will take care of the rest.
Summary
If you're already using webpack, strongly consider implementing the Brotli compression plugin to further reduce the size of your bundles. Implementing this into your workflow should only take a couple of minutes and with nearly global browser support, there is hardly a reason not to anymore. Unless of course, you're using a proxy server or CDN which doesn't support Brotli-compressed assets. However, with KeyCDN this is as easy as enabling the Cache Brotli feature in your Zone settings.
Simply ensure that your origin server supports the delivery of Brotli assets, and your users will begin receiving smaller-sized assets instantly.