Tips for Improving CSS and JS Animation Performance
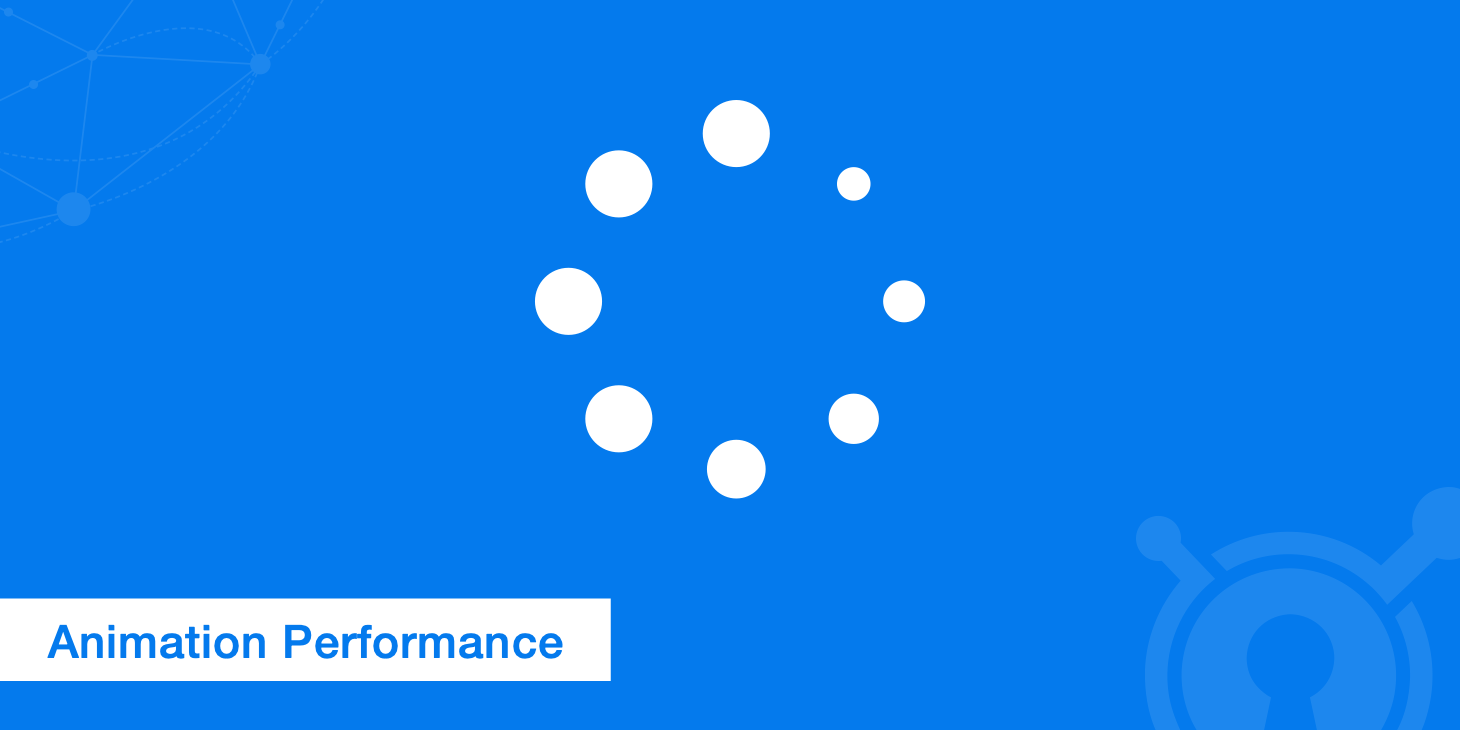
Custom animations can help a website stand out among the competition, but poorly optimized assets can lead to performance issues that ultimately drive away users. If any of your animations render at less than 60 frames per second, then your visitors will notice, and the user experience will suffer. This guide explains how to keep your CSS and JavaScript animations running smoothly for everyone.
CSS animations vs JavaScript animations
Animations made with JavaScript are sometimes called imperative animations, and those made with CSS are called declarative animations. CSS animations are handled by the browser's compositor thread rather than the main thread responsible for painting and styling. Consequently, such animations are unaffected by the main thread's more expensive tasks. Of course, animations that trigger the paint or layout events will require work from the main thread, which negates the benefits of using CSS animations.
JavaScript, on the other hand, always gets executed by the main thread. Nonetheless, JavaScript gives you greater control over animations, so you're better off using it in some situations. While CSS is ideal for simple transitions, JavaScript is recommended for animations with advanced effects such as bouncing. Animations that allow the user to pause or rewind should also be animated with JavaScript. JavaScript is necessary to implement certain effects like parallax scrolling. With the help of the Web Animations API, you can create complex animations for object-oriented applications. You can also use JavaScript to control CSS animations.
JavaScript animation tips
1. Use requestAnimationFrame()
Instead of using setTimeOut()
and setInterval()
, use the native JavaScript method requestAnimationFrame()
to execute your animation code at the best time for the browser. This method chooses the appropriate frame rate for the user's device, so mobile visitors will see a different frame rate than desktop users.
2. Decouple animations from events
The code responsible for handling events such as scrolling should be kept separate from your animation code. HTML5Rocks has a great write up on this which dives deeper into how you should decouple events from the code that handles requestAnimationFrame()
.
3. Keep your JavaScript code concise
Be wary of adding huge chunks of JavaScript to your web page. If your code becomes unwieldy, you could try using web workers to execute JavaScript animations on a different thread.
4. Don't use jQuery
Stay away from older animation libraries like jQuery. If you're looking for something similar, Velocity.js works basically the same way, but it's much faster and supports more features. Replacing jQuery's $.animate() with $.velocity() will make a major difference for mobile users. Web Designer Depot has a nearly exhaustive list of animation tools for JavaScript.
Choosing which CSS properties to animate
Continuous animations can consume a significant amount of resources, but some CSS properties are more costly to animate than others. The harder a browser must work to animate a property, the slower the frame rate will be. Therefore, choosing the right CSS properties to animate can have a huge impact on page performance.
There are three main types of CSS properties:
- Layout properties - These determine the size and placement of page elements. Animations that change an element's width and height can affect the placement of other page elements, which can cause a chain reaction known as "layout thrash." Since animations that change the page layout are especially costly, they are best avoided.
- Paint properties - These define the appearance of page elements. Making changes to properties such as color requires repainting, which can be costly. That said, simple animations that require a small portion of the viewport to be repainted may have a negligible impact on overall performance. Larger animations that require repainting may not be worth the effort.
- Composite properties - Which include transform and opacity, are your best friends for creating CSS animations with minimal cost. With transform, you can scale and rotate animations without affecting the page layout. Try to use composite properties for animations whenever possible. With a little creative thinking, you'd be surprised by what you can pull off with just these properties.
For best results, try to limit yourself to using these four composite properties:
- position
- scale
- rotation
- opacity
You can do almost everything using these four options that you can using layout properties without affecting the positioning of other page elements. For example, to change the size of an image, use scale() rather than width().
CSS animation tips
1. Avoid simultaneous animations
Animations that run smoothly in isolation may not work so well on a page alongside dozens of other animations. More than two animations going at the same time is likely to cause lag. Therefore, timing your animations so that they don't all execute all at once is vital to maintaining consistent performance. This can be accomplished by adding transition delays. Figuring out how to optimally choreograph your animations may require some trial and error, but the performance boost you'll get is worth the effort.
2. Examine your animations in slow motion
If an animation looks good in slow motion, it will look great at normal speed. Slowing down an animation can help you better pinpoint rendering problems.
3. Delay all animations by a fraction of a second
Since the browser is very busy when your page begins loading, delaying all animations until a few hundred milliseconds after the initial load event can make a noticeable difference in overall page performance.
4. Don't bind CSS animations to scroll
Animations that follow the viewport as the user scrolls are not only annoying, but they also drag down the performance of everything else on screen.
5. Combine CSS with SVGs
Scalable vector graphics, or SVGs, are excellent for animations since they can be scaled without degrading resolution. You can create SVGs in a program like Adobe Illustrator and apply CSS to alter their appearance. Just another reason to choose SVGs over icon fonts.
When to use will-change
If you've tried everything else and are still having performance problems, then you might want to try adding the will-change
property to your animations. As its name implies, will-change indicates that an element's properties will change so that the browser can make appropriate preparations. List the specific properties that will change like so:
.element {
will-change: transform, opacity;
}
Since using will-change
consumes resources, be warned that overuse can lead to further performance problems. Putting it before every animation by default isn't recommended. Only use will-change
as a last resort to optimize page performance.
Put your animations to the test
Performance testing should be an ongoing process while building animations for your web app. The longer you wait to identify rendering issues, the harder it becomes to pinpoint the root of the problem.
The developer tools for Chrome, Firefox and Safari offer a frame-by-frame breakdown of paint and render events under their Network tabs. This information can help you optimize animations as you design them. If you're using Chrome, look under the Rendering tab in the DevTools console for additional features such as an FPS meter.
The user's screen size has a major impact on how animations display, so be sure to test your project on multiple platforms including mobile devices.
Summary
Optimizing animations for the web is a relatively straight-forward process. Choosing engaging animations that will entice new visitors to stay on your website is more challenging because you have to consider your audience. In addition to tracking how your site's technical performance affects conversions, take note of the impact that web animations have on your bottom line. If your animations aren't helping, then they are just wasting resources better spent elsewhere.